DECENTRALIZED APP DEVELOPMENT MADE EASY
All your core functions in one place.
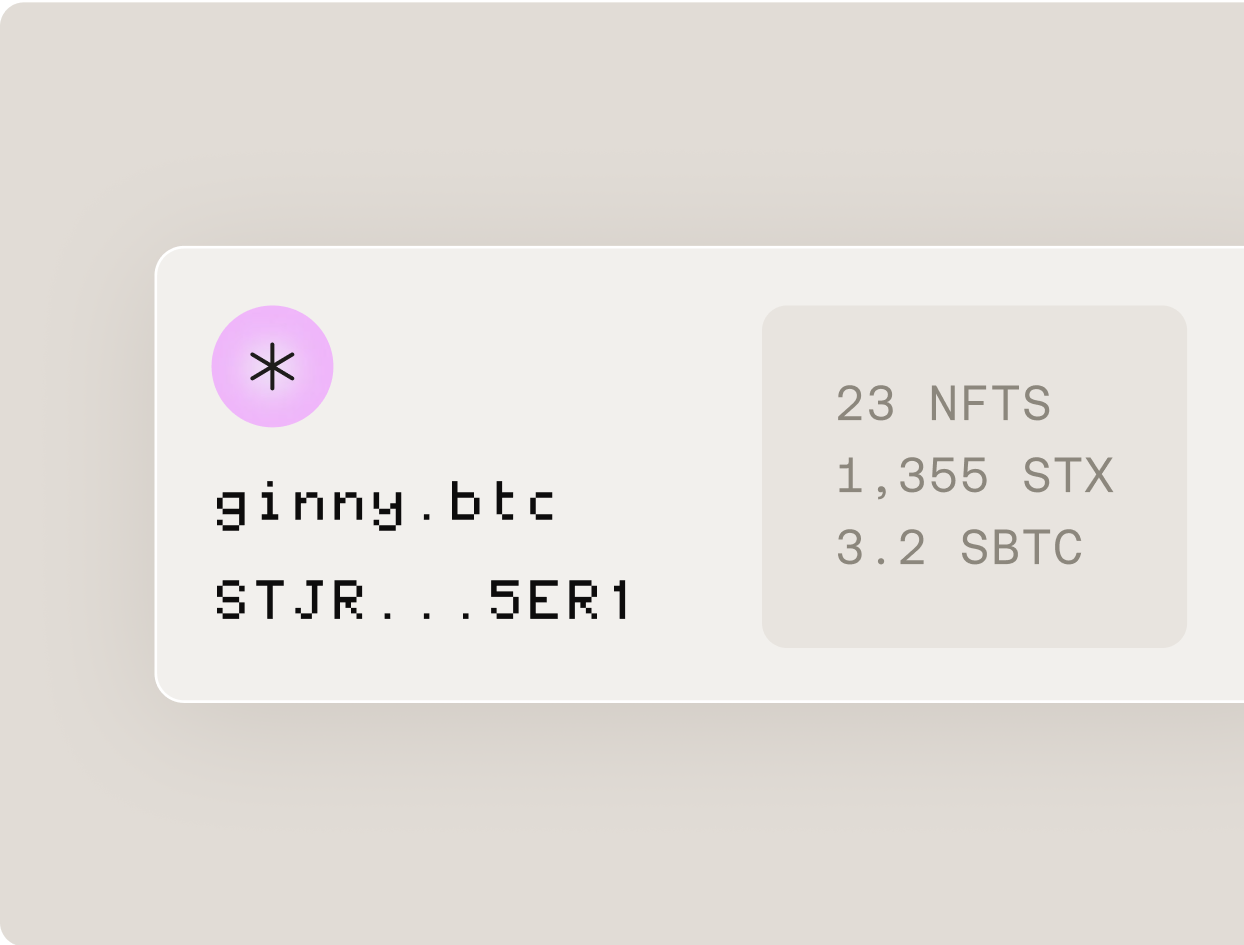
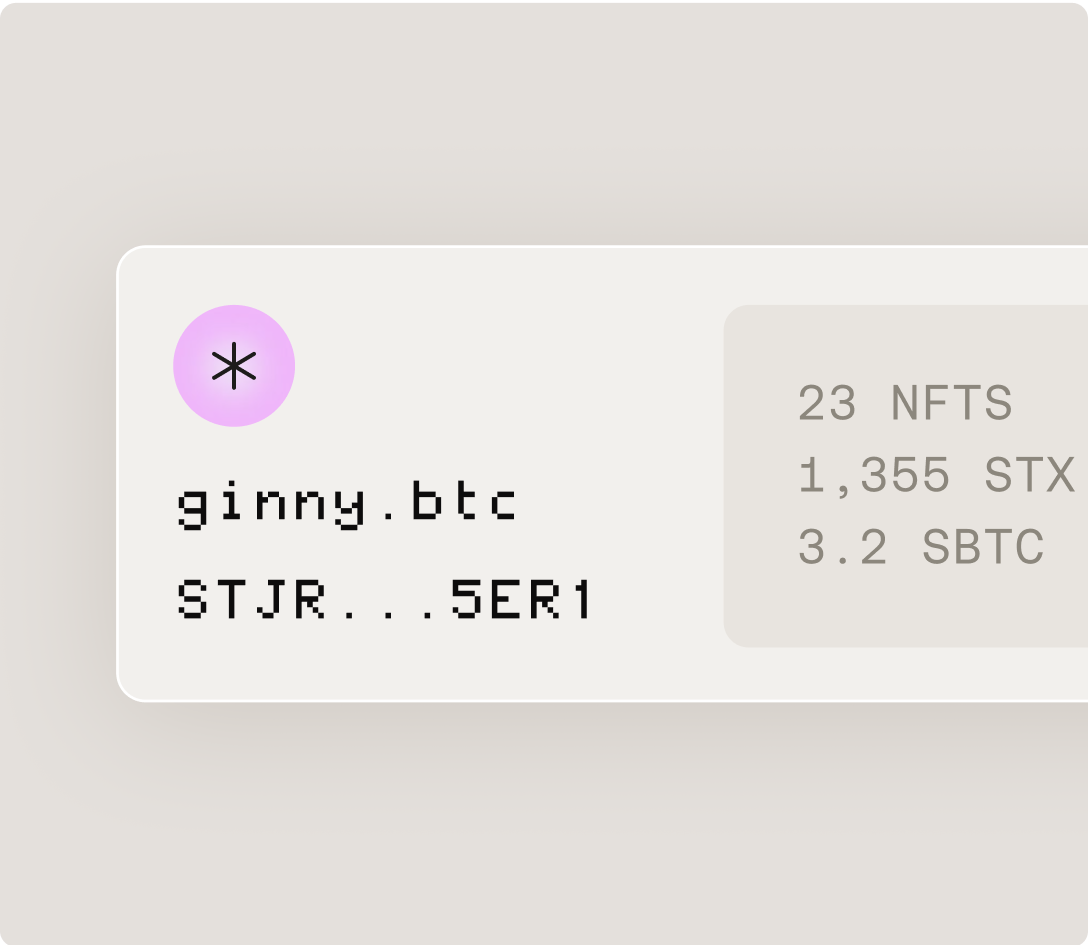
Authenticate your users
Connect to user wallets and verify their on-chain identity.
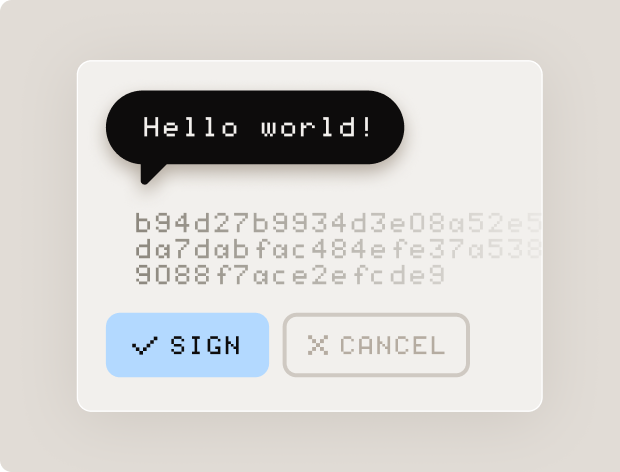
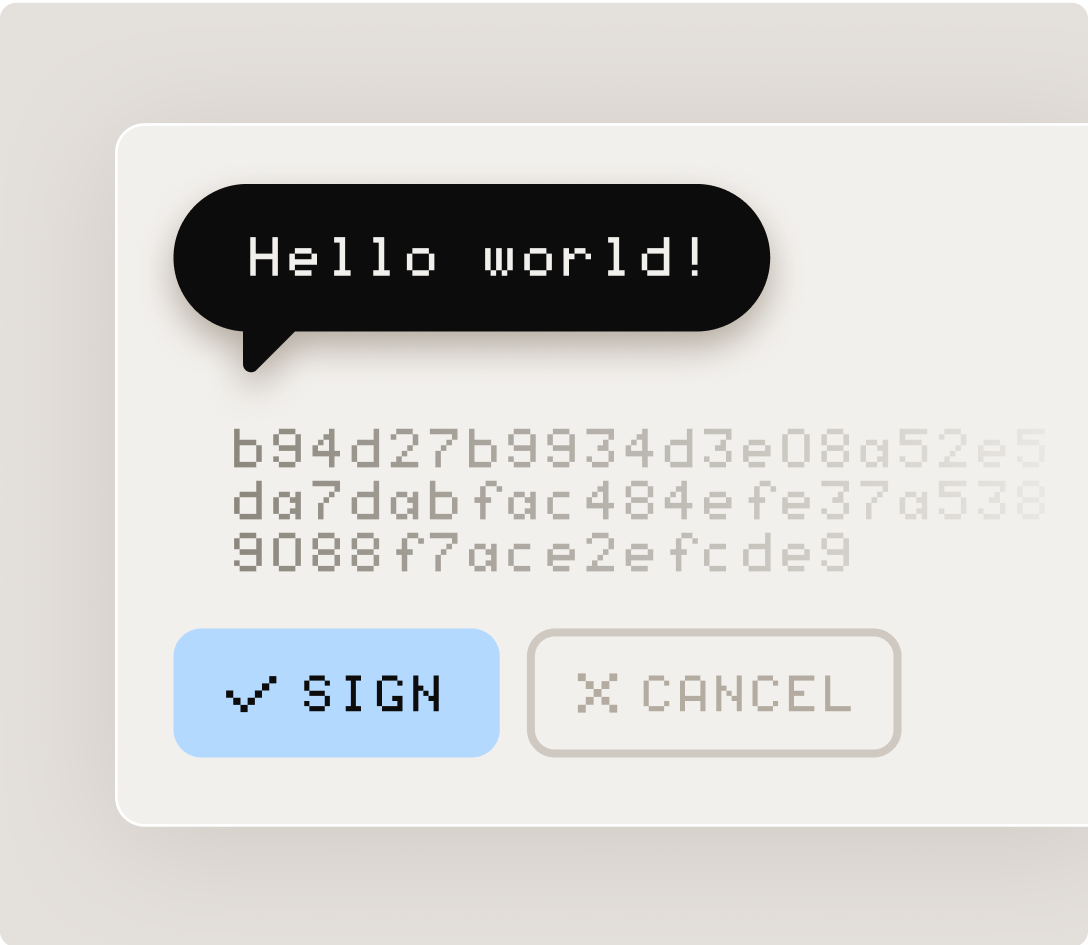
Sign messages
Prompt users to sign arbitrary messages, which can authorize an in-app action or prove they control a particular address.
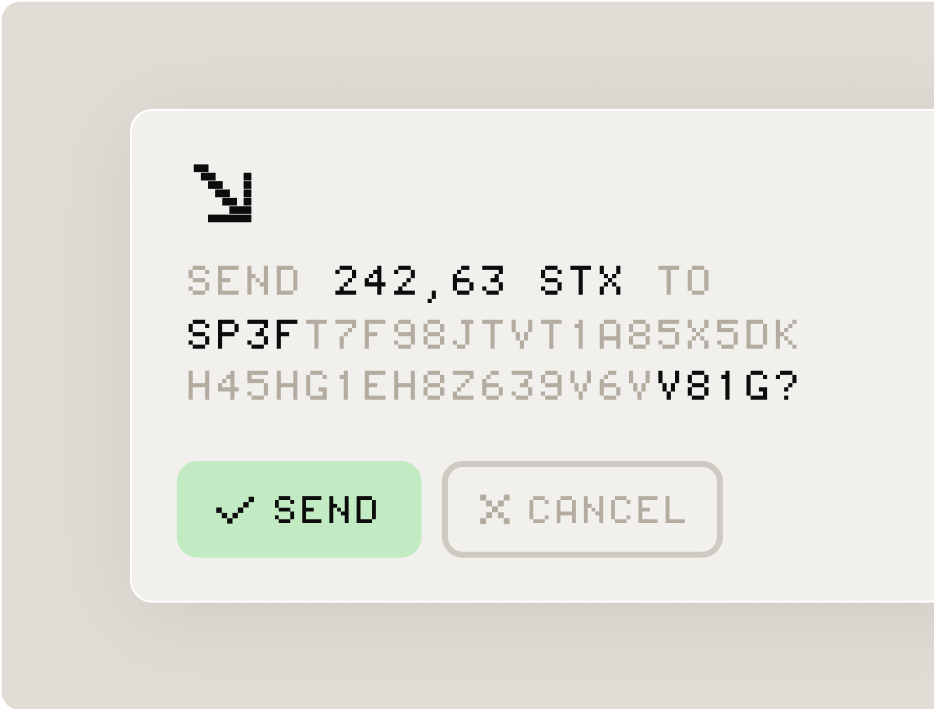

Sign transactions
Enable your users to interact with smart contracts through your app.
/** Stacks.js is the building block that we use all the time to build, broadcast, and sign our transactions. */
Take advantage of what Stacks has to offer.
Create post conditions
Stacks.js also enables you to create new post conditions, a unique safety feature of Stacks. Post conditions are requirements a smart contract transaction needs to meet in order to execute (otherwise it will abort). Those requirements could be a minimum/maximum transfer amount from the sender, what the receiver will send back in return, and more.
Stacks Connect
Leverage the Stacks Connect library to authenticate your users, enabling them to log in to your app. This package also handles broadcasting in-app transactions to the Stacks network, a critical step in putting user actions on-chain. Connect your app to the network with one of the most popular packages of Stacks.js.